C# Sample
This code is for an application that can store songs with a title, artist, mood, and rating to a database. The database can then be searched by title, artist, mood or rating. The application also allows the user to use his own SQL queries for a more refined search. This application also employs OpenGL for the graphical text display at the top of the application.
See the code sample and screenshot below.
SongSearch.CS Sample:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.SqlServerCe;
using OpenTK.Graphics;
using OpenTK.Graphics.OpenGL;
using QuickFont;
namespace SongSearch
{
public partial class Form1 : Form
{
private DataSet ds = new DataSet("SongList");
private SqlCeDataAdapter dAdapter;
private DataView dataView;
private DataTable dTable;
private int _selectedIndex=-1;
private bool _loaded = false;
private QFont _font1;
private QFont _font2;
public Form1()
{
InitializeComponent();
string cnStr = @"Data Source=SongList.sdf";
dAdapter = new SqlCeDataAdapter("Select * From Songs", cnStr);
SqlCeCommandBuilder cb = new SqlCeCommandBuilder();
cb.DataAdapter = dAdapter;
dAdapter.Fill(ds, "Songs");
dAdapter.Update(ds.Tables[0]);
dataGridView1.DataSource = ds.Tables[0];
this.glControl1.Load += new System.EventHandler(this.glControl1_Load);
this.glControl1.Paint += new System.Windows.Forms.PaintEventHandler(this.glControl1_Paint);
this.glControl1.Resize += new System.EventHandler(this.glControl1_Resize);
}
private void glControl1_Paint(object sender, PaintEventArgs e)
{
if (!_loaded) // Play nice
return;
GL.Clear(ClearBufferMask.ColorBufferBit | ClearBufferMask.DepthBufferBit);
GL.MatrixMode(MatrixMode.Modelview);
GL.LoadIdentity();
Render();
glControl1.SwapBuffers();
}
private void glControl1_Load(object sender, EventArgs e)
{
GL.ClearColor(Color.SkyBlue);
SetupViewport();
Application.Idle += Application_Idle; // press TAB twice after +=
_font1 = new QFont("../../Fonts/HappySans.ttf", 36, new QFontBuilderConfiguration(true));
_font1.Options.Colour = new Color4(0.8f, 0.2f, 0.2f, 1.0f);
_font2 = new QFont("../../Fonts/HappySans.ttf", 30, new QFontBuilderConfiguration(true));
_font2.Options.Colour = new Color4(0.0f, 0.0f, 0.0f, 1.0f);
_loaded = true;
}
void Application_Idle(object sender, EventArgs e)
{
if(_loaded)
glControl1.Invalidate();
}
private void glControl1_Resize(object sender, EventArgs e)
{
if (!_loaded)
return;
SetupViewport();
glControl1.Invalidate();
}
private void SetupViewport()
{
int w = glControl1.Width;
int h = glControl1.Height;
GL.MatrixMode(MatrixMode.Projection);
GL.LoadIdentity();
GL.Ortho(0, w, 0, h, -1, 1); // Bottom-left corner pixel has coordinate (0, 0)
GL.Viewport(0, 0, w, h); // Use all of the glControl painting area
}
private void Render()
{
string songTitle = "Dream On";
songTitle = textBoxTitle.Text;
QFont.Begin();
GL.PushMatrix();
GL.Translate(339, 0, 0);
_font1.Print(songTitle, QFontAlignment.Centre);
GL.PopMatrix();
GL.PushMatrix();
GL.Translate(339, 50, 0);//x was 281
_font2.Print(textBoxBand.Text, QFontAlignment.Centre);
GL.PopMatrix();
QFont.End();
GL.Color3(Color.Blue);
}
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
if (e.RowIndex == -1)
{
return;
}
else
{
_selectedIndex = e.RowIndex;
fillForm(e.RowIndex);
}
}
private void fillForm(int selectedIndex)
{
labelSongID.Text = dataGridView1.Rows[selectedIndex].Cells[6].Value.ToString();
numericUpDownRating.Value = (int)dataGridView1.Rows[selectedIndex].Cells[2].Value;
textBoxTitle.Text = dataGridView1.Rows[selectedIndex].Cells[0].Value.ToString();
textBoxBand.Text = dataGridView1.Rows[selectedIndex].Cells[1].Value.ToString();
textBoxGenre.Text = dataGridView1.Rows[selectedIndex].Cells[3].Value.ToString();
textBoxMood.Text = dataGridView1.Rows[selectedIndex].Cells[4].Value.ToString();
textBoxTopic.Text = dataGridView1.Rows[selectedIndex].Cells[5].Value.ToString();
}
private void btnAdd_Click(object sender, EventArgs e)
{
DataRow songRow = ds.Tables[0].NewRow();
songRow["Title"] = textBoxTitle.Text;
songRow["Band"] = textBoxBand.Text;
songRow["Rating"] = numericUpDownRating.Value;
songRow["Genre"] = textBoxGenre.Text;
songRow["Mood"] = textBoxMood.Text;
songRow["Topic"] = textBoxTopic.Text;
try
{
ds.Tables[0].Rows.Add(songRow);
dAdapter.Update(ds.Tables[0]);
}
catch (Exception ex)
{
}
}
private void button1_Click(object sender, EventArgs e)
{
_selectedIndex = -1;
string cnStr = @"Data Source=SongList.sdf";
dAdapter = new SqlCeDataAdapter("Select * From Songs", cnStr);
SqlCeCommandBuilder cb = new SqlCeCommandBuilder();
cb.DataAdapter = dAdapter;
ds.Clear();
dAdapter.Fill(ds, "Songs");
dataGridView1.DataSource = ds.Tables[0];
}
private void btnUpdate_Click(object sender, EventArgs e)
{
if (_selectedIndex == -1) return;
ds.Tables[0].Rows[_selectedIndex]["Title"] = textBoxTitle.Text;
ds.Tables[0].Rows[_selectedIndex]["Band"] = textBoxBand.Text;
ds.Tables[0].Rows[_selectedIndex]["Rating"] = numericUpDownRating.Value;
ds.Tables[0].Rows[_selectedIndex]["Genre"] = textBoxGenre.Text;
ds.Tables[0].Rows[_selectedIndex]["Mood"] = textBoxMood.Text;
ds.Tables[0].Rows[_selectedIndex]["Topic"] = textBoxTopic.Text;
try
{
dAdapter.Update(ds.Tables[0]);
}
catch (Exception ex)
{
}
}
private void btnDelete_Click(object sender, EventArgs e)
{
if (_selectedIndex == -1) return;
try
{
ds.Tables[0].Rows[_selectedIndex].Delete();
dAdapter.Update(ds.Tables[0]);
}
catch (Exception ex)
{
}
}
private void btnSearch_Click(object sender, EventArgs e)
{
string str = "";
string searchStr = "Select * From Songs where Rating >= ";
_selectedIndex = -1;
string cnStr = @"Data Source=SongList.sdf";
searchStr += numericUpDownSRating.Value.ToString();
if (textBoxSTitle.TextLength > 0)
{
str = " and Title Like '%" + textBoxSTitle.Text + "%'";
searchStr += str;
}
if (textBoxSBand.TextLength > 0)
{
str = " and Band Like '%" + textBoxSBand.Text + "%'";
searchStr += str;
}
if (textBoxSTopic.TextLength > 0)
{
str = " and Topic Like '%" + textBoxSTopic.Text + "%'";
searchStr += str;
}
if (textBoxSGenre.TextLength > 0)
{
str = " and Genre Like '%" + textBoxSGenre.Text + "%'";
searchStr += str;
}
if (textBoxSMood.TextLength > 0)
{
str = " and Mood Like '%" + textBoxSMood.Text + "%'";
searchStr += str;
}
textBoxSS.Text = searchStr;
try
{
dAdapter = new SqlCeDataAdapter(searchStr, cnStr);
SqlCeCommandBuilder cb = new SqlCeCommandBuilder();
cb.DataAdapter = dAdapter;
ds.Clear();
dAdapter.Fill(ds, "Songs");
dataGridView1.DataSource = ds.Tables[0];
}
catch (Exception ex)
{
}
}
private void btnClear_Click(object sender, EventArgs e)
{
textBoxSTitle.Text = "";
textBoxSBand.Text = "";
textBoxSTopic.Text = "";
textBoxSGenre.Text = "";
textBoxSMood.Text = "";
}
private void btnSQL_Click(object sender, EventArgs e)
{
string searchStr;// = "Select * From Songs where Rating >= ";
_selectedIndex = -1;
string cnStr = @"Data Source=SongList.sdf";
searchStr = textBoxSS.Text;
try
{
dAdapter = new SqlCeDataAdapter(searchStr, cnStr);
SqlCeCommandBuilder cb = new SqlCeCommandBuilder();
cb.DataAdapter = dAdapter;
ds.Clear();
dAdapter.Fill(ds, "Songs");
dataGridView1.DataSource = ds.Tables[0];
}
catch (Exception ex)
{
}
}
private void Form1_Load(object sender, EventArgs e)
{
int test=1;
test = 2;
}
}
}
Screenshot of Song Search Application. (click on image to expand)
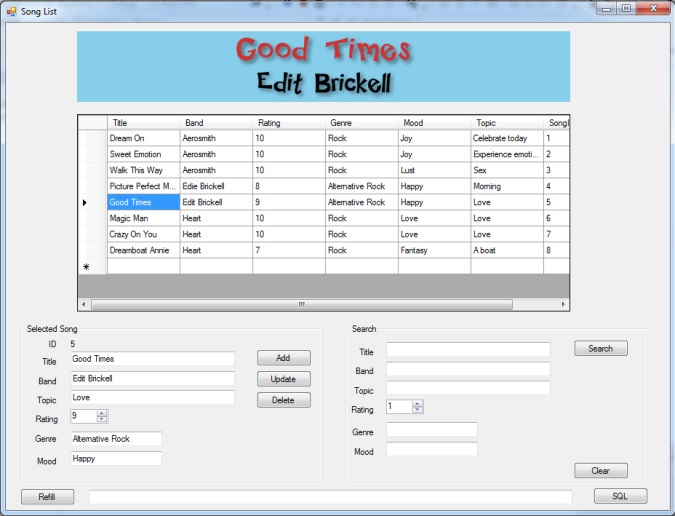